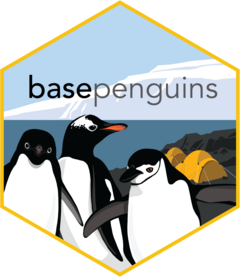
Convert files to use datasets versions of penguins and penguins_raw
Source:R/convert.R
convert_files.Rd
These functions convert files that use the
palmerpenguins package
to use the versions of penguins
and penguins_raw
included in the datasets
package in R >= 4.5.0. They removes calls to library(palmerpenguins)
and make
necessary changes to some variable names (see Details section below).
Arguments
- input
For
convert_files()
andconvert_files_inplace()
: A character vector of file paths to convert. Can be relative or absolute paths. Forconvert_dir()
andconvert_dir_inplace()
: A string with a path to a directory of files to convert.- output
For
convert_files()
: A character vector of output file paths, orNULL`` to modify files in place. If provided, must be the same length as
input. Can be absolute or relative paths. For
convert_dir(): A string with the output directory, or
NULL` to modify the files in the directory in place.- extensions
A character vector of file extensions to process, defaults to R scripts and R Markdown and Quarto documents.
Value
A list (returned invisibly) with two components:
changed
: A character vector of paths for files that were modified.not_changed
: A character vector of paths for files that were not modified. Files are not changed if they do not load the palmerpenguins package vialibrary(palmerpenguins)
,library('palmerpenguins')
orlibrary("palmerpenguins")
, or if they do not have one of the specifiedextensions
.
For both the changed
and not_changed
vectors, these will be subsets of
the output
paths, if they were provided, with the corresponding input
paths as names. If output
was not specified, then these vectors will be
subsets of the input
paths, and the vectors will not be named.
Details
Files are converted by:
Replacing
library(palmerpenguins)
with the empty string""
Replacing
data("penguins", package = "palmerpenguins")
withdata("penguins", package = "datasets")
Replacing variable names:
bill_length_mm
->bill_len
bill_depth_mm
->bill_dep
flipper_length_mm
->flipper_len
body_mass_g
->body_mass
Replacing
ends_with("_mm")
withstarts_with("flipper_"), starts_with"("bill_")
Non-convertible files (those without the specified extensions) are copied to
the output location if output
is provided, but are not modified.
If the output
files or directory do not (yet) exist, they will be created
(recursively if necessary).
Replacing ends_with("_mm")
with starts_with("flipper_"), starts_with("bill_")
ensures that modified R code will always run. starts_with("flipper_")
isn't
intuitively necessary, as there is only one variable starting with "flipper_",
in penguins
, but this code will not error inside dplyr::(select)
, even if
flipper_len
isn't in the data frame (trying to select flipper_len
directly will cause an error if that column isn't in the data frame).
In an educational context, we suggest manually editing the converted files to
replace starts_with("flipper_")
to flipper_len
if appropriate.
To facilitate this, the functions documented here produce a message
indicating the files and line numbers where the ends_with("_mm")
substitution was made.
Examples
# Note that all examples below write output to a temporary directory
# and file paths are relative to that directory.
# For all examples below, use a copy of the examples provided by the package,
# copied to an "examples" directory in the working directory
example_dir("examples")
# Single file - new output
result <- convert_files("examples/penguins.R", "penguins_new.R")
#> - ends_with("_mm") replaced on line 7 in penguins_new.R
#> - Please check the changed output files.
cat(readLines("penguins_new.R"), sep = "\n") # view changes
#>
#> library(ggplot2)
#> library(dplyr)
#>
#> # exploring scatterplots
#> penguins |>
#> select(body_mass, starts_with("flipper_"), starts_with("bill_")) |>
#> ggplot(aes(x = flipper_len, y = body_mass)) +
#> geom_point(aes(color = species, shape = species), size = 2) +
#> scale_color_manual(values = c("darkorange", "darkorchid", "cyan4"))
# Single file - copy, then modify that in place
file.copy("examples/penguins.R", "penguins_copy.R")
#> [1] TRUE
convert_files_inplace("penguins_copy.R")
#> - ends_with("_mm") replaced on line 7 in penguins_copy.R
#> - Please check the changed output files.
# Multiple files - new output locations
input_files <- c("examples/penguins.R", "examples/nested/penguins.qmd")
output_files <- output_paths(input_files, dir = "new_dir")
convert_files(input_files, output_files)
#> - ends_with("_mm") replaced on line 7 in new_dir/examples/penguins_new.R
#> - Please check the changed output files.
#> - Remember to re-knit or re-render and changed Rmarkdown or Quarto documents.
# Directory - new output location
result <- convert_dir("examples", "new_directory")
#> - ends_with("_mm") replaced on line 7 in new_directory/penguins.R
#> - Please check the changed output files.
#> - Remember to re-knit or re-render and changed Rmarkdown or Quarto documents.
result # see `changed` and `not_changed` files
#> $changed
#> examples/nested/penguins.qmd examples/penguins.R
#> "new_directory/nested/penguins.qmd" "new_directory/penguins.R"
#>
#> $not_changed
#> examples/no_penguins.Rmd examples/nested/not_a_script.md
#> "new_directory/no_penguins.Rmd" "new_directory/nested/not_a_script.md"
#>
# Overwrite the files in "examples"
result <- convert_dir_inplace("examples")
#> - ends_with("_mm") replaced on line 7 in examples/penguins.R
#> - Please check the changed output files.
#> - Remember to re-knit or re-render and changed Rmarkdown or Quarto documents.
result # see `changed` and `not_changed` files
#> $changed
#> [1] "examples/nested/penguins.qmd" "examples/penguins.R"
#>
#> $not_changed
#> [1] "examples/no_penguins.Rmd" "examples/nested/not_a_script.md"
#>